Are you ready to explore the captivating realm of data structures and algorithms in Python? This extensive guide is designed for both novices and seasoned programmers, providing you with the essential knowledge and skills to tackle intricate problems, enhance code efficiency, and fully harness Python’s capabilities.
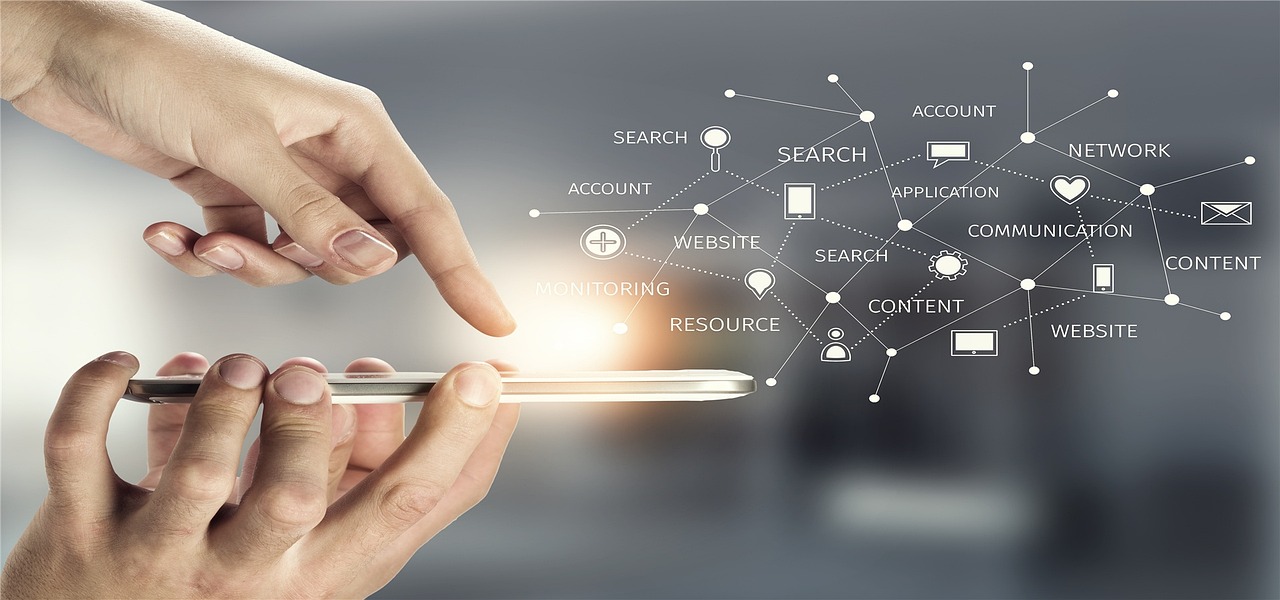
In this article, we will delve into the core principles of data structures and algorithms, examine their significance in software development, and discover effective ways to implement them using Python. Prepare yourself for an engaging journey into the world of data structures and algorithms!
Introduction
In today’s digital landscape, the ability to process and manipulate data is crucial across numerous fields. From analyzing extensive datasets to optimizing algorithms, a solid understanding of data structures and algorithms is essential for any programmer. Data structures serve as frameworks for organizing and storing data, while algorithms facilitate efficient operations and problem-solving.Python has emerged as a favored language for implementing these concepts due to its simplicity and adaptability. With a rich array of built-in data structures and an extensive library ecosystem, Python empowers developers to confront a wide variety of computational challenges effectively.This guide will delve into the fundamental data structures and algorithms in Python, outlining their characteristics, practical applications, and implementation strategies. Whether you are just starting or have experience in programming, this article aims to equip you with the knowledge and tools necessary to fully utilize data structures and algorithms in Python.
Understanding Data Structures
Before we explore specific data structures, it’s important to grasp what they are and their significance in software development. In programming, data structures are specialized formats for organizing and storing data, enabling efficient access, manipulation, and storage. This organization allows programmers to write optimized and maintainable code.The selection of a data structure is influenced by the specific problem being solved and the operations required on the data. Different structures excel in various scenarios; for instance, if you need an unordered collection of elements, a list may be appropriate. Conversely, if you need rapid access to data via unique keys, a dictionary would be more suitable.
Data structures can be categorized into two main types: primitive and abstract. Primitive data structures include basic types such as integers, floats, and booleans that are built into the language for storing individual values. Abstract data structures are more complex constructs composed of multiple elements, defined by the programmer using primitive types.
Python offers a diverse set of built-in data structures including lists, tuples, dictionaries, sets, and strings. Furthermore, both the standard library and third-party packages provide specialized data structures tailored to specific needs. By mastering these structures and understanding their properties, you’ll be better prepared to address various programming challenges efficiently.
Lists: The Swiss Army Knife of Data Structures
Lists are often regarded as one of the most versatile data structures in programming, particularly in Python. They serve as an essential tool for organizing and manipulating data, making them invaluable in various applications.
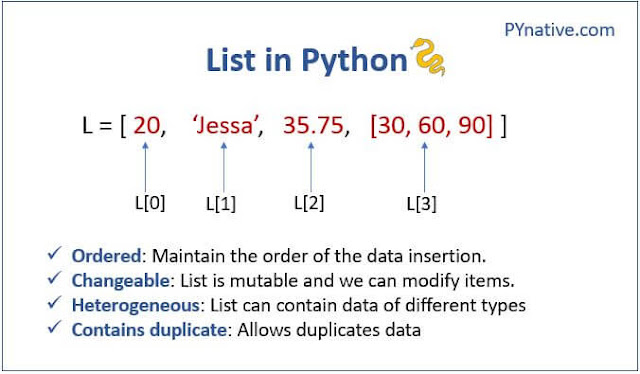
IMAGE SOURCE:-PYnative.com
What is a List?
A list can be defined as an abstract data type that stores elements in an ordered manner, allowing for efficient retrieval and manipulation. Unlike arrays, which typically hold homogeneous data types, lists can contain heterogeneous items, meaning they can store different types of data within the same structure14.
Key Characteristics
- Dynamic Size: Lists in Python are dynamic, meaning their size can grow or shrink as needed without requiring a predefined limit. This flexibility allows developers to add or remove elements easily1.
- Ordered Collection: Elements in a list maintain the order in which they were added, enabling easy access through indexing. For example, the first element is accessed with index 01.
- Allow Repetition: Lists can contain duplicate values. Each entry is treated as a distinct item, which is particularly useful when the same data needs to be represented multiple times1.
Common Operations on Lists
Python lists support a variety of operations that make them easy to use:
- Creation: Lists can be created using square brackets. For example:python
my_list = [1, 2, 3, 'a', 'b']
- Accessing Elements: Elements are accessed using their index:python
first_element = my_list[0] # Output: 1
- Updating Elements: You can change the value of an element by assigning a new value to its index:python
my_list[0] = 'changed'
- Appending Elements: New elements can be added to the end of the list using the
append()
method:pythonmy_list.append(4)
- Removing Elements: Elements can be removed using methods like
remove()
orpop()
:pythonmy_list.remove('a')
Use Cases
Lists are particularly useful in scenarios where:
- You need to maintain an ordered collection of items.
- The data may change frequently (adding or removing elements).
- You require a flexible structure that can handle various data types.
Tuples: Immutable Containers
Tuples are a fundamental data structure in Python, known for their immutability and versatility. They provide a way to store an ordered collection of items, making them a useful tool in various programming scenarios.
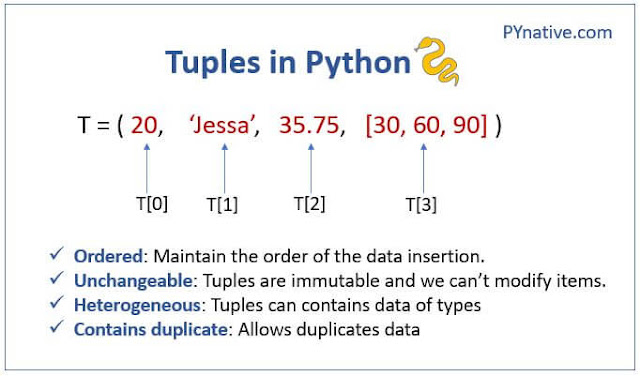
IMAGE SOURCE:-PYnative.com
What is a Tuple?
A tuple is an ordered collection of elements that can be of any data type. Unlike lists, which are mutable and can be modified after creation, tuples are immutable, meaning that once they are defined, their contents cannot be altered. This immutability makes tuples particularly useful in situations where a constant set of values is needed13.
Key Characteristics
- Ordered: Tuples maintain the order of elements based on their insertion sequence, allowing for easy indexing.
- Immutable: Once a tuple is created, its elements cannot be changed, added, or removed. This property ensures that the data remains constant throughout the program’s execution24.
- Heterogeneous: Tuples can store items of different data types within the same structure, including integers, strings, and even other tuples34.
- Memory Efficient: Due to their fixed size and immutability, tuples generally consume less memory compared to lists. This makes them more efficient for certain applications3.
Creating and Accessing Tuples
Tuples can be created using parentheses ()
or the tuple()
constructor. Here are some examples:
python# Creating a tuple using parentheses
my_tuple = (1, 2, 3)
# Creating a tuple using the tuple() constructor
another_tuple = tuple([4, 5, 6])
Elements in a tuple can be accessed using indexing:
pythonfirst_element = my_tuple[0] # Output: 1
Common Operations on Tuples
While tuples do not support methods that modify their contents (like append
or remove
), they do support several operations:
- Concatenation: You can combine two tuples to create a new one:python
combined_tuple = my_tuple + another_tuple # Output: (1, 2, 3, 4, 5, 6)
- Repetition: You can repeat the contents of a tuple:python
repeated_tuple = my_tuple * 2 # Output: (1, 2, 3, 1, 2, 3)
- Membership Testing: Use the
in
operator to check if an element exists in a tuple:pythonexists = (2 in my_tuple) # Output: True
Use Cases for Tuples
Tuples are particularly beneficial in scenarios where:
You require a lightweight structure for storing data that will not change.
You need to ensure that data remains unchanged throughout the program.
You want to represent fixed collections of items such as coordinates (e.g., (x, y)
), RGB color values (255, 0, 0)
, or records like (name, age)
in a database4.
Dictionaries: Efficient Key-Value Mapping in Python
Dictionaries are a powerful and flexible data structure in Python, designed for storing data in key-value pairs. This unique mapping allows for efficient data retrieval and manipulation, making dictionaries an essential tool for many programming tasks.
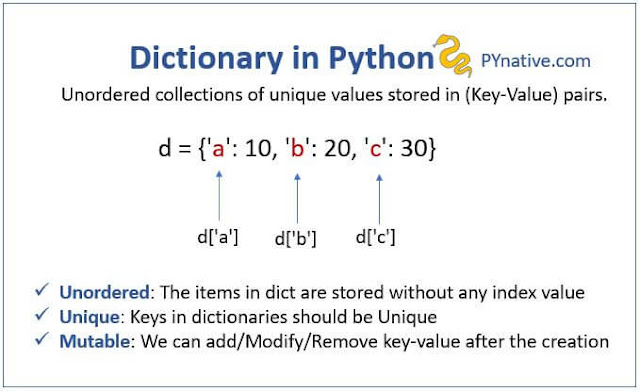
IMAGE SOURCE:-PYnative.com
What is a Dictionary?
A dictionary in Python is an unordered collection of items where each item consists of a key and its corresponding value. The keys act as unique identifiers for the values, enabling fast access and retrieval.
Key Characteristics
- Unordered: Unlike lists or tuples, dictionaries do not maintain the order of elements. The keys are stored in a hash table, which allows for quick lookups.
- Mutable: Dictionaries can be modified after creation. You can add, remove, or change key-value pairs dynamically.
- Unique Keys: Each key in a dictionary must be unique; however, multiple keys can map to the same value.
Creating a Dictionary
Dictionaries can be created using curly braces {}
or the dict()
constructor. Here are some examples:
python# Creating a dictionary using curly braces
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Creating a dictionary using dict()
another_dict = dict([('apple', 1), ('banana', 2), ('cherry', 3)])
Accessing Values
You can retrieve values from a dictionary using their corresponding keys:
pythonvalue = my_dict['banana'] # Output: 2
To avoid errors when accessing non-existent keys, you can use the get()
method:
pythonvalue = my_dict.get('orange', 'Not Found') # Output: 'Not Found'
Common Operations on Dictionaries
- Adding or Updating Key-Value Pairs: You can add new pairs or update existing ones using square brackets:
pythonmy_dict['orange'] = 4 # Adds a new key-value pair
my_dict['apple'] = 5 # Updates the existing key
- Removing Key-Value Pairs: Use the
del
statement or thepop()
method to remove items:
pythondel my_dict['banana'] # Removes 'banana' from the dictionary
value = my_dict.pop('cherry') # Removes 'cherry' and returns its value
- Iterating Over Dictionaries: You can iterate through keys, values, or key-value pairs using methods like
.keys()
,.values()
, and.items()
:
pythonfor key in my_dict.keys():
print(key)
for value in my_dict.values():
print(value)
for key, value in my_dict.items():
print(f"{key}: {value}")
Use Cases for Dictionaries
Dictionaries are particularly useful in scenarios such as:
- Storing related data pairs (e.g., user profiles with usernames as keys).
- Implementing fast lookups where quick access to data is essential (e.g., caching results).
- Representing structured data like JSON objects or configuration settings.
Sets: Unordered Unique Elements
Sets are a fundamental data structure in Python, designed to store unordered collections of unique elements. Their primary characteristics make them particularly useful for various programming tasks, especially those involving membership testing and eliminating duplicates.
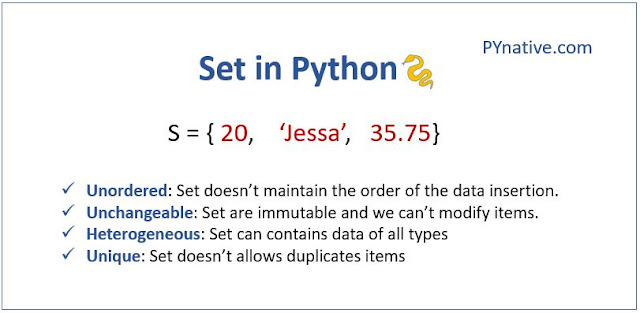
IMAGE SOURCE:-PYnative.com
What is a Set?
A set is an unordered collection that contains only unique elements. This means that no two elements in a set can be the same, and any attempt to add duplicates will be ignored. Sets are mutable, allowing for the addition and removal of elements, but the individual elements themselves must be of an immutable type (e.g., integers, strings, tuples).
Key Characteristics
- Unordered: The elements in a set do not have a specific order. When you print a set, the order of the items may vary.
- Unique Elements: Sets automatically handle duplicates; any duplicate entries are disregarded.
- Mutable: While the contents of a set can change (you can add or remove items), you cannot modify an element directly once it is added.
- Hashable Elements: Only hashable types can be included in a set. This means you cannot add lists or dictionaries as elements.
Creating Sets
Sets can be created using curly braces {}
or the built-in set()
function. Here are examples of both methods:
python# Creating a set using curly braces
fruits = {'apple', 'banana', 'orange'}
# Creating a set using the set() constructor
numbers = set([1, 2, 3, 4])
Common Operations on Sets
- Adding Elements: Use the
add()
method to include new items:pythonfruits.add('grape') # Adds 'grape' to the set
- Removing Elements: You can remove items using
discard()
orremove()
. The difference is thatdiscard()
does not raise an error if the item does not exist:pythonfruits.discard('banana') # Removes 'banana' if it exists
- Popping Elements: The
pop()
method removes and returns an arbitrary element from the set:pythonitem = fruits.pop() # Removes and returns an arbitrary fruit
- Membership Testing: Check if an element exists in a set using the
in
keyword:pythonexists = 'apple' in fruits # Returns True if 'apple' is present
Use Cases for Sets
Sets are particularly advantageous in scenarios such as:
- Eliminating Duplicates: Quickly remove duplicate entries from collections.
- Membership Testing: Efficiently check for the existence of an element.
- Mathematical Set Operations: Perform operations like union, intersection, and difference between sets.
Arrays: Efficient Numeric Computation
Arrays are a fundamental data structure in programming, particularly suited for efficient numeric computation. They allow for the storage of a fixed number of values in contiguous memory locations, making them optimal for scenarios where performance and memory efficiency are crucial.

IMAGE SOURCE:-PYnative.com
What is an Array?
An array is a collection of elements stored at contiguous memory locations. This structure enables fast access to elements via their indices, making operations like reading and writing data efficient. In Python, while arrays are not natively supported as a distinct data type, they can be implemented using libraries such as array
or NumPy
.
Key Characteristics
- Fixed Size: Arrays have a predetermined size, which means the number of elements must be known in advance.
- Contiguous Memory Allocation: Elements are stored in adjacent memory locations, allowing for quick access and manipulation.
- Efficient Indexing: Accessing an element by its index is a constant time operation, denoted as O(1)O(1).
Creating and Using Arrays
In Python, you can create arrays using the array
module or the more powerful NumPy
library. Here’s how to do it:
Using the array
Module
pythonimport array
# Creating an array of integers
int_array = array.array('i', [1, 2, 3, 4, 5])
Using NumPy
NumPy provides a more versatile and efficient way to handle arrays:
pythonimport numpy as np
# Creating a NumPy array
np_array = np.array([1, 2, 3, 4, 5])
Common Operations on Arrays
Arrays support various operations that enhance their utility in numeric computations:
- Accessing Elements: Retrieve values using indices.
pythonvalue = np_array[0] # Output: 1
- Updating Elements: Modify values at specific indices.
pythonnp_array[0] = 10
- Slicing: Extract subsets of the array.
pythonsubset = np_array[1:3] # Output: array([2, 3])
- Mathematical Operations: NumPy allows for vectorized operations across entire arrays.
pythonsquared = np_array ** 2 # Output: array([100, 4, 9, 16, 25])
Advantages of Using Arrays
- Memory Efficiency: Arrays consume less memory compared to lists when storing large amounts of data of the same type due to their contiguous storage.
- Performance: Operations on arrays are generally faster than those on lists because of their fixed size and contiguous nature.
- Numerical Computation: Libraries like NumPy offer optimized functions for complex mathematical calculations, making them ideal for scientific computing and data analysis.
Use Cases for Arrays
Arrays are particularly beneficial in scenarios such as:
- Storing large datasets where the size is known ahead of time (e.g., temperature readings over a month).
- Performing mathematical computations efficiently (e.g., statistical analysis).
- Implementing algorithms that require fast access to elements (e.g., sorting or searching).
Strings: Immutable Sequence of Characters
Strings in Python are a fundamental data structure that represents an immutable sequence of characters. This immutability is a key feature that distinguishes strings from other data types, such as lists.
What is a String?
A string is a collection of characters enclosed in quotes (single, double, or triple). Strings can include letters, numbers, symbols, and whitespace.
Key Characteristics
- Immutable: Once a string is created, its content cannot be changed. Any operation that modifies a string will result in the creation of a new string rather than altering the original.
- Ordered: Strings maintain the order of characters, allowing for indexing and slicing.
- Iterable: Strings can be iterated over, enabling operations like looping through each character.
Understanding Immutability
The immutability of strings means that you cannot modify an existing string directly. For example:
pythongreeting = "Hello"
greeting[0] = "J" # This will raise a TypeError
Instead, if you want to change a string, you must create a new one:
pythonnew_greeting = "J" + greeting[1:] # Output: "Jello"
This behavior ensures that strings remain consistent and predictable throughout their use in a program.
Benefits of Immutability
- Hashability: Immutable objects can be used as keys in dictionaries because their hash value remains constant. This property is essential for maintaining consistent key-value mappings.
- Memory Efficiency: Python can optimize memory usage by reusing immutable objects instead of creating new ones each time.
- Thread Safety: Immutability provides inherent thread safety. Multiple threads can access the same string without risk of data corruption due to concurrent modifications.
- Predictability: With immutable strings, you can trust that the value of a string will not change unexpectedly, leading to easier debugging and maintenance.
Common String Operations
- Concatenation: You can combine strings using the
+
operator:pythonfull_greeting = greeting + ", world!" # Output: "Hello, world!"
- Slicing: Extracting parts of a string can be done using slicing:python
substring = greeting[1:4] # Output: "ell"
- String Methods: Python provides numerous built-in methods for string manipulation, such as
.upper()
,.lower()
,.replace()
, and more:pythonupper_case = greeting.upper() # Output: "HELLO"
Use Cases for Strings
Strings are widely used in various applications such as:
- Storing textual data (e.g., names, addresses).
- Representing user input in applications.
- Working with data formats like JSON or XML.
Data Structures and Algorithms in Python: Frequently Asked Questions (FAQs)
Understanding data structures and algorithms is crucial for any programmer, especially when working with Python. Below are some frequently asked questions that can help clarify key concepts and practices related to data structures and algorithms in Python.
1. What are Data Structures?
Data structures are specialized formats for organizing, processing, and storing data efficiently. They enable programmers to manage data in a way that optimizes performance based on the specific needs of an application.
2. What are the Commonly Used Data Structures in Python?
The most common data structures in Python include:
- Lists: Ordered and mutable collections.
- Tuples: Ordered and immutable collections.
- Dictionaries: Unordered collections of key-value pairs.
- Sets: Unordered collections of unique elements.
- Arrays: Fixed-size collections of items of the same type.
3. What Operations Can Be Performed on Data Structures?
Common operations include:
- Insertion: Adding elements.
- Deletion: Removing elements.
- Traversal: Accessing each element.
- Searching: Finding specific elements.
- Sorting: Arranging elements in a specific order.
4. How Do Lists Differ from Arrays?
While both lists and arrays can store collections of items, lists are dynamic and can hold mixed data types, whereas arrays (typically from the array
module or NumPy
) are fixed-size and require all elements to be of the same type.
5. What is a Dictionary?
A dictionary is an unordered collection of key-value pairs, where each key must be unique. It allows for fast lookups, insertions, and deletions based on keys.
6. What Are Tuples Used For?
Tuples are immutable collections that can store heterogeneous data types. They are often used for fixed collections of items, such as coordinates or multiple return values from functions.
7. What Are Sets and Their Use Cases?
Sets are unordered collections of unique elements, making them ideal for membership testing and eliminating duplicate entries. They support operations like union, intersection, and difference.
8. How Do You Choose the Right Data Structure?
Choosing the right data structure depends on:
- The type of operations you need to perform (e.g., searching vs. sorting).
- The nature of the data (e.g., whether duplicates are allowed).
- Performance requirements (e.g., memory usage vs. speed).
9. What is an Algorithm?
An algorithm is a step-by-step procedure or formula for solving a problem or performing a task. It typically involves a series of well-defined instructions to achieve a desired outcome.
10. Why is Python Popular for Data Structures and Algorithms?
Python’s simplicity and readability make it an excellent choice for learning and implementing data structures and algorithms. Its rich set of built-in data types and libraries (like NumPy
for arrays) further enhance its capabilities in this area.By familiarizing yourself with these concepts, you can effectively utilize data structures and algorithms in your Python programming endeavors, leading to more efficient and effective code solutions.
Conclusion
In conclusion, mastering data structures and algorithms is essential for any programmer looking to enhance their coding skills and problem-solving abilities. Python, with its rich set of built-in data types and libraries, provides an excellent platform for implementing these concepts effectively.
Key Takeaways:
- Understanding Data Structures: Familiarity with various data structures—such as lists, tuples, dictionaries, sets, and arrays—enables you to choose the right tool for the task at hand. Each structure has unique characteristics that make it suitable for specific scenarios.
- Importance of Algorithms: Algorithms are the backbone of efficient programming. Knowing how to implement and optimize algorithms can significantly improve the performance of your applications.
- Practical Application: The real-world application of data structures and algorithms extends beyond theoretical knowledge. Engaging in coding challenges, projects, and practical scenarios will solidify your understanding and enhance your skills.
- Continuous Learning: The field of computer science is ever-evolving. Staying updated with new developments, best practices, and advanced techniques will keep you competitive and proficient in your programming journey.
By leveraging the power of data structures and algorithms in Python, you can write more efficient, maintainable, and scalable code. Whether you are a beginner or an experienced developer, investing time in mastering these concepts will undoubtedly pay off in your programming career. Happy coding!
0 Comments