JavaScript offers a variety of methods for displaying output, including console.log()
, alert()
, document.write()
, and direct manipulation of HTML elements. Each method serves specific purposes, such as debugging, notifying users, or dynamically updating web content.
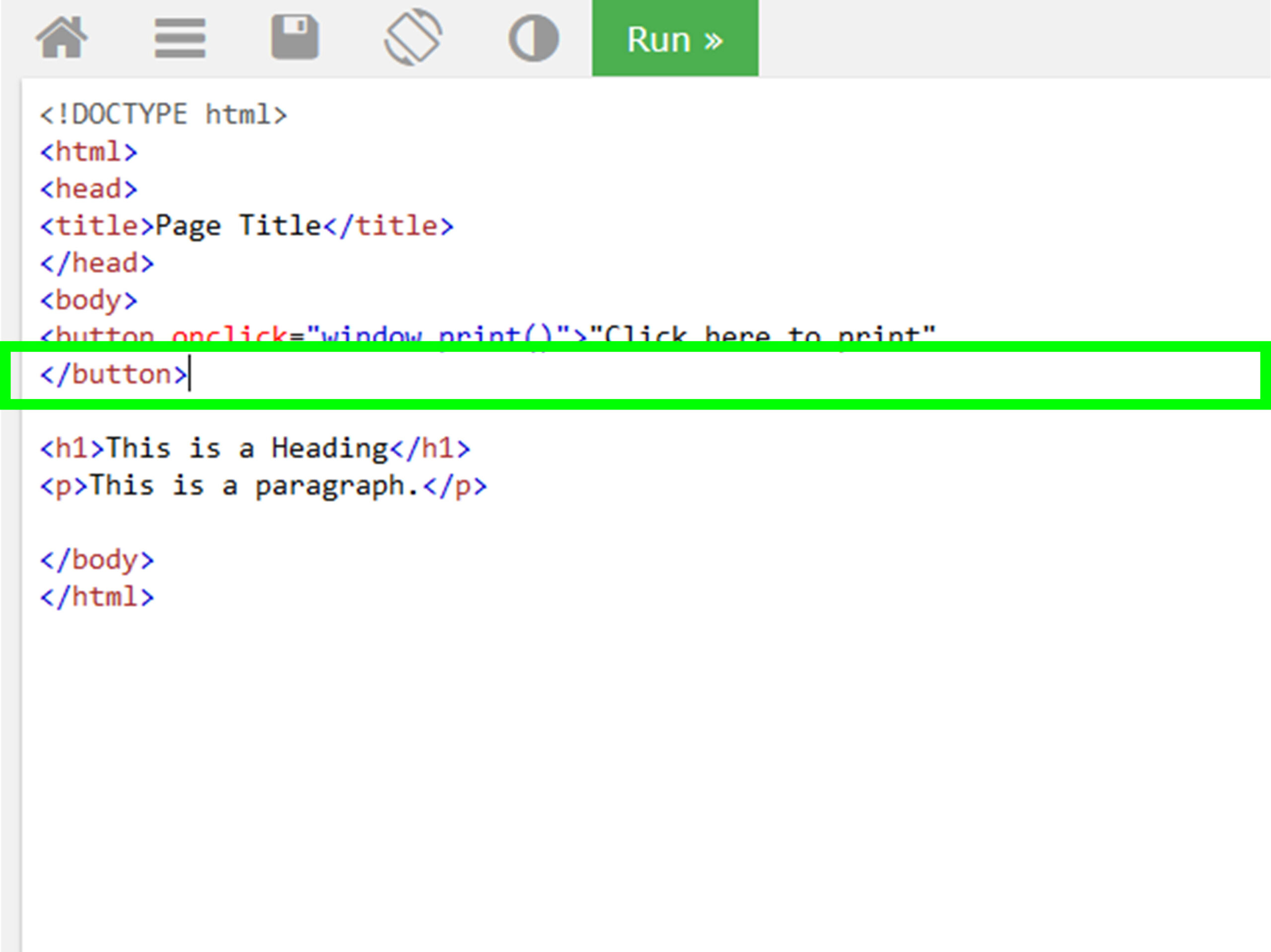
Overview of JavaScript Output Methods
console.log()
: Primarily used for debugging, this method outputs messages to the browser’s console. It is not visible on the webpage, making it ideal for developers to test and log information without disturbing the user experience.alert()
: This method creates a pop-up alert box that displays a message to the user. While useful for important notifications, it interrupts the user’s interaction with the page until dismissed, so it should be used sparingly.document.write()
: This method writes directly to the HTML document. However, it is generally discouraged in modern web development because using it after the document has loaded can overwrite existing content. It is more suitable for simple demonstrations or testing rather than production use.- Manipulating HTML Elements: Using methods like
innerHTML
, developers can update specific parts of a webpage dynamically. This approach is preferred for creating interactive and responsive web applications.
Recommendations
While there are various methods to display output in JavaScript, it’s advisable to focus on console.log()
for debugging and innerHTML
for updating content dynamically. The use of alert()
should be limited due to its intrusive nature, and document.write()
is best avoided in favor of more modern techniques that promote better separation of concerns in web development.
Summary Table of Output Methods
Method | Purpose | Example Code |
---|---|---|
console.log() | Debugging messages | console.log("Message"); |
alert() | Display alert dialog | alert("Message"); |
document.write() | Write directly to HTML | document.write("Content"); |
innerHTML | Modify HTML element content | document.getElementById("id").innerHTML = "Content"; |
window.prompt() | Capture user input | let input = prompt("Enter something"); |