Embarking on the journey to master data structures is akin to unlocking a new level of coding expertise, essential for anyone venturing into algorithm design. This advanced data structures tutorial serves as your guide through the intricate landscape of programming, helping you organize and manipulate data with both precision and efficiency. Whether you’re just starting out in algorithm design or seeking to enhance your existing skills, this guide is crafted to illuminate your path.
Our comprehensive tutorial simplifies the complexities of data structures, breaking down sophisticated concepts into easily digestible components. It caters not only to beginners but also provides valuable insights for experienced programmers, featuring clear, step-by-step instructions alongside real-world scenarios that bridge theory and practice. From the elegance of well-crafted algorithms to the robust frameworks of data structures, this tutorial aims to inspire and empower you, laying a solid foundation for innovation and problem-solving in your coding endeavors.
Letâs embark on this transformative journey together, enhancing your understanding of data structures in Java and elevating your programming skills to new heights.
Common Data Structures in Java
Let’s explore some of the most commonly used data structures in Java:
1. Arrays
Arrays in Java function like organized shelves in a library, efficiently storing elements of the same type for quick access. They are fundamental data structures, allowing programmers to retrieve each element using a simple index. For beginners, mastering arrays is essential for understanding Java’s data management capabilities.Creating an array in Java is straightforward:
javaint[] numberList = new int[5]; // Initializes an array to hold five integers
This code snippet marks your initial foray into managing multiple data points effectively. Arrays serve as the foundation for more complex data structures, facilitating the development of efficient and robust Java applications.
2. Linked List
The linked list is a dynamic collection of nodes, where each node connects to the next, creating a flexible structure for data management. Unlike arrays, linked lists can grow and shrink in size as needed, making them ideal for situations where memory allocation is unpredictable. A notable feature of linked lists is their ability to reverse direction, allowing traversal from the end back to the beginning.Hereâs how to create a linked list in Java:
javaLinkedList<String> myList = new LinkedList<>();
myList.add("First");
myList.add("Second");
myList.add("Third");
This code snippet initiates your journey into linked lists, with each add
method call representing a new node being added to the list. Understanding linked lists goes beyond simply learning about a data structure; it encourages you to think about adaptable and efficient data handling that evolves alongside your program’s requirements.
3. Queue Data Structure
The queue data structure embodies the âFirst In First Outâ (FIFO) principle, resembling real-world scenarios such as lines at a ticket counter or task scheduling systems. This structure ensures that the first element added to the queue will be the first one to be removed, promoting orderly processing of data.To initiate a queue in Java, you can use:
javaQueue<String> customerQueue = new LinkedList<>();
customerQueue.add("Customer1");
customerQueue.add("Customer2");
customerQueue.add("Customer3");
This block of code sets up a system that prioritizes fairness and efficiency. The versatility of queues makes them applicable in various scenarios, including resource allocation and managing asynchronous tasks across software applications. Embracing queues in programming fosters solutions that reflect systematic organization, ensuring that every piece of data is handled with care and timeliness.
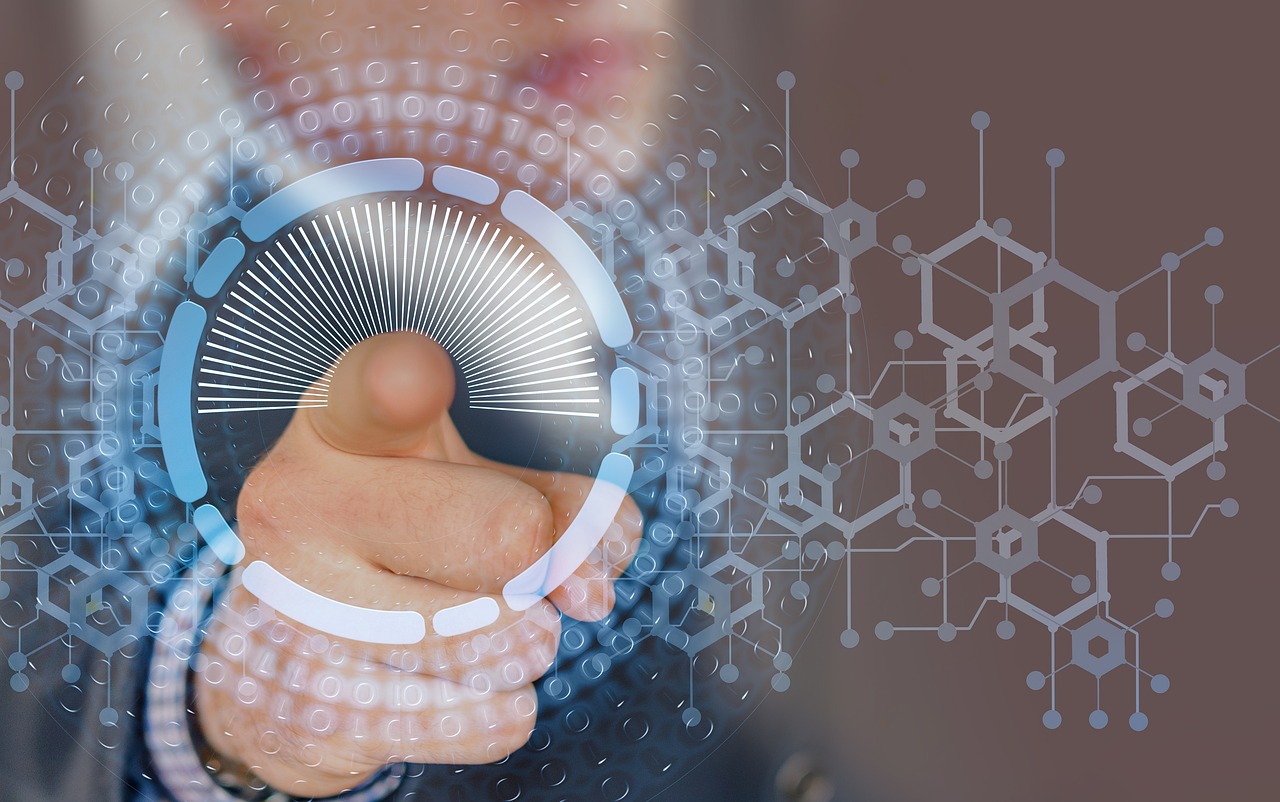
Applications of Queue Data Structure
The queue data structure is a fundamental component in computer science, widely utilized across various applications due to its efficient management of data in a First In First Out (FIFO) manner. Here are some key applications:
Task Scheduling
Queues are instrumental in managing tasks that need to be executed sequentially. They ensure that each task is processed in the order it arrives or according to priority, effectively streamlining workflows and enhancing productivity.
Resource Allocation
Queues play a crucial role in the fair distribution of limited resources, such as CPU time or printer access. By organizing requests in a queue, systems can maximize resource utilization while minimizing wait times for users.
Batch Processing
In scenarios involving large sets of tasksâlike data analysis or renderingâqueues help organize and manage these jobs systematically. This approach prevents system overload and allows for efficient processing without overwhelming resources.
Message Buffering
In communication networks, queues temporarily store messages to ensure smooth and orderly transmission between senders and receivers. This buffering is vital for maintaining data integrity and flow in communications.
Event Handling
For applications that react to user actions or system events, queues help manage these events sequentially. This organization ensures a responsive and intuitive user experience, allowing systems to handle multiple inputs efficiently.
Traffic Management
Queues are utilized in various traffic control systems, from air traffic management to roadway systems. They help organize the flow of vehicles, reducing congestion and improving safety by methodically managing movement sequences.
Operating Systems
Operating systems leverage queues for process scheduling, where programs wait their turn to utilize system resources. This organization promotes fairness and efficiency in multitasking environments.
Network Protocols
In networking, protocols like TCP use queues for packet management, ensuring that data packets are transmitted and received in the correct order. This is crucial for maintaining data integrity across network communications.Each application of the queue data structure highlights its versatility and efficiency in organizing, managing, and processing data across a wide range of real-world scenarios, demonstrating the power of structured programming in addressing everyday challenges.
Stack Data Structure
The stack data structure operates on the principle of Last In First Out (LIFO), resembling a neatly stacked pile of plates. This structure ensures that the last element added is the first to be removed, making it ideal for scenarios such as undo operations in software or navigating browser histories.
Applications of Stack Data Structure
Stacks are not just for simple data storage; they play a vital role in algorithm implementation. For instance:
- Expression Parsing: Stacks are used to evaluate expressions in calculators by managing operators and operands.
- Function Call Management: They track active function calls in programming languages, enabling features like recursion.
Creating a Stack in Java:
javaStack<Integer> myStack = new Stack<>();
myStack.push(10);
myStack.push(20);
myStack.pop();
This code snippet illustrates how stacks operate, demonstrating their capability to add and remove elements efficiently. The stack’s simplicity makes it an invaluable tool for enhancing code clarity and functionality across various applications.
Tree Data Structure
The tree data structure represents hierarchical relationships akin to a natural tree with branches extending from a single root. It organizes data across multiple levels, facilitating efficient data management and operations essential for algorithms and software applications.
Applications of Tree Data Structure
Trees are foundational in many computing areas:
- Database Management Systems: Trees enable rapid searches and organized storage.
- Network Routing Algorithms: They facilitate efficient data routing across networks.
Basic Tree Structure in Java:
javaclass Node {
String name;
Node[] children;
Node(String name) {
this.name = name;
this.children = new Node[0]; // Initially no children
}
}
This code snippet initiates a simple tree node that can be expanded into complex structures. Trees provide a flexible approach to organizing and accessing data while mirroring real-life complexities.
Graphs
Graphs consist of vertices (or nodes) connected by edges, serving as powerful tools for modeling complex relationships. Their non-linear structure excels at representing networksâfrom social connections to computer networksâoffering both visual and conceptual frameworks for understanding interactions.
Applications of Graph Data Structure
Graphs have diverse applications:
- Route Optimization: Used in logistics to find the most efficient paths.
- Social Network Analysis: Helps analyze relationships within social platforms.
Creating a Simple Graph in Java:
javaimport java.util.*;
class Graph {
private Map<Integer, List<Integer>> adjList;
public Graph() {
adjList = new HashMap<>();
}
public void addEdge(int start, int end) {
adjList.computeIfAbsent(start, x -> new ArrayList<>()).add(end);
adjList.computeIfAbsent(end, x -> new ArrayList<>()).add(start);
}
}
This snippet lays the groundwork for exploring the interconnected nature of graphs. Their ability to map connections makes them indispensable for solving complex problems efficiently across various domains.
Navigating the landscape of technical interviews can be challenging, especially when it comes to data structure interview questions. These questions not only assess your knowledge but also provide an opportunity to demonstrate your problem-solving abilities and understanding of fundamental concepts. Whether you’re an experienced Java developer or a newcomer, familiarity with key data structure interview questions can give you a competitive edge. Here are some common questions and their answers to help you prepare effectively.
1. What is a Data Structure?
A data structure is a systematic way of organizing, managing, and storing data so that it can be accessed and modified efficiently. The choice of data structure depends on the operations required for the data.
2. Can you explain the difference between an array and a linked list?
Arrays and linked lists are both used to store collections of data. An array stores elements in contiguous memory locations, allowing for fast access via indexes, but its size is fixed once declared. In contrast, a linked list consists of nodes that are not stored contiguously; each node points to the next one, allowing for dynamic resizing but slower access to elements.Java Code Snippet for Array:
javaint[] myArray = {1, 2, 3, 4, 5};
Java Code Snippet for Linked List:
javaLinkedList<String> myLinkedList = new LinkedList<>();
myLinkedList.add("First");
myLinkedList.add("Second");
3. What is a Stack, and where is it used?
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. It functions like a stack of plates where only the top plate can be added or removed. Stacks are commonly used in function call management in programming languages, undo mechanisms in applications, and evaluating expressions.
4. Describe a Queue and its applications.
A queue is another linear data structure that operates on the First In, First Out (FIFO) principle, similar to a line of people waiting for service. Queues are used in task scheduling, managing requests on shared resources (like printers), and implementing breadth-first search algorithms.
5. How would you reverse a linked list in Java?
Reversing a linked list involves changing the direction of the pointers so that the first node becomes the last and vice versa.Java Code Snippet:
javapublic ListNode reverseLinkedList(ListNode head) {
ListNode prev = null;
ListNode current = head;
while (current != null) {
ListNode nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
6. What are the applications of the tree data structure?
Trees are non-linear data structures ideal for representing hierarchical relationships. They are extensively used in databases for indexing, in compilers for syntax trees, and for managing hierarchical data such as file systems. Diving into these data structure interview questions not only prepares you for technical interviews but also deepens your understanding of efficient data management and manipulation techniques. Each question provides an opportunity to showcase your analytical skills and enthusiasm for technology. Approach them with confidence to let your knowledge shine through.
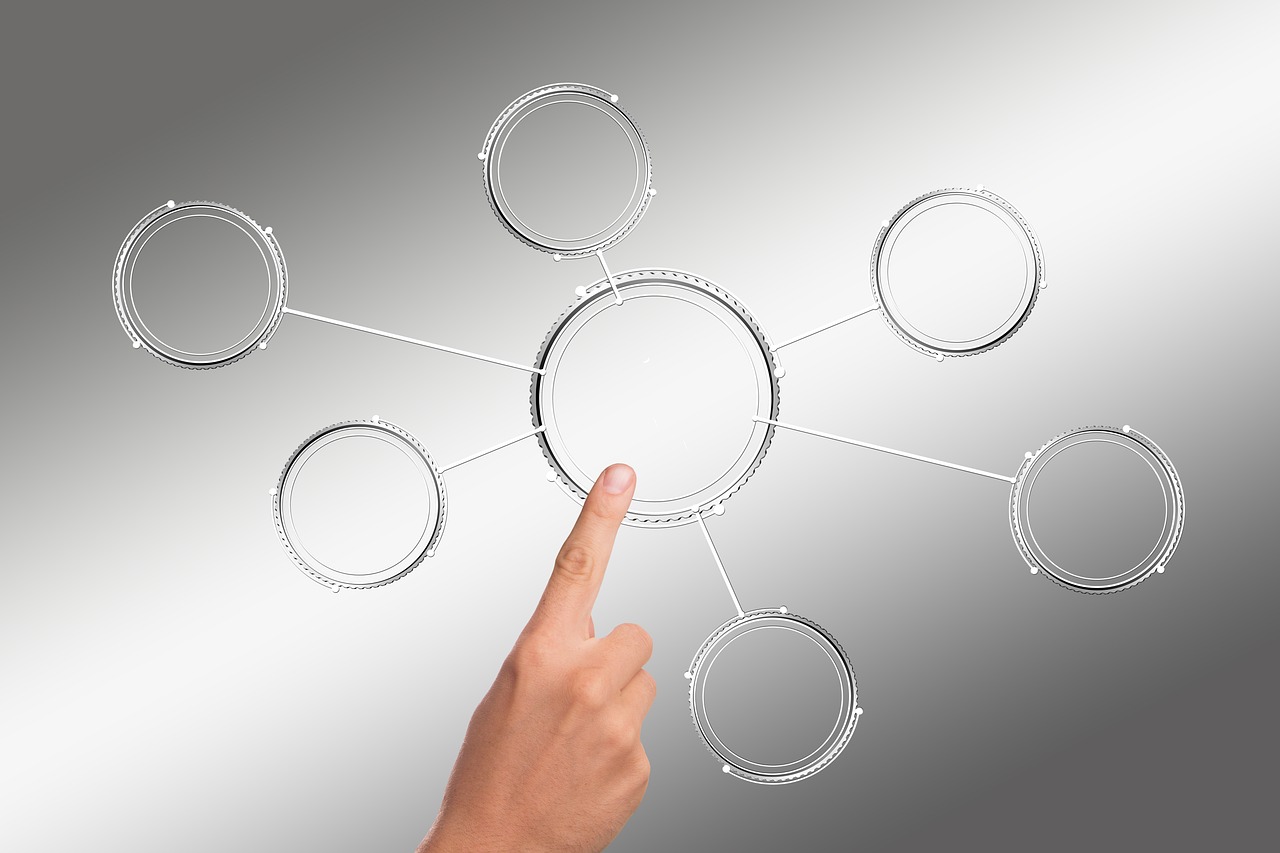
uestion | Answer |
---|---|
1. What is a Data Structure? | A data structure is a systematic way to organize, manage, and store data to enable efficient access and modification. |
2. Difference between an Array and a Linked List? | – Array: Stores elements in contiguous memory locations, allowing fast access via indexes; size is fixed upon declaration. – Linked List: Comprises nodes that are not stored contiguously; allows dynamic resizing but has slower access to elements. |
3. What is a Stack, and where is it used? | A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. Itâs used in function call management, undo mechanisms, and evaluating expressions. |
4. Describe a Queue and its applications. | A queue operates on the First In, First Out (FIFO) principle. Itâs used in task scheduling, managing requests on shared resources (like printers), and breadth-first search algorithms. |
5. How would you reverse a linked list in Java? | Reversing a linked list involves changing the direction of the pointers so that the first node becomes the last.java<br>public ListNode reverseLinkedList(ListNode head) {<br> ListNode prev = null;<br> ListNode current = head;<br> while (current != null) {<br> ListNode nextTemp = current.next;<br> current.next = prev;<br> prev = current;<br> current = nextTemp;<br> }<br> return prev;<br>}<br> |
6. Applications of the Tree Data Structure? | Trees are non-linear structures ideal for hierarchical relationships, used in databases for indexing, compilers for syntax trees, and managing hierarchical data like file systems. |
7. What is a Postfix Expression? | A postfix expression is one where operators follow their operands, eliminating the need for parentheses to denote operation precedence. |
8. Applications of Data Structures? | Used in database management, AI and machine learning, operating systems, compilers, and graphics processing. |
9. Explain Linear vs Non-Linear Data Structures. | – Linear Data Structures: Elements arranged sequentially (e.g., arrays, linked lists). – Non-Linear Data Structures: Elements arranged hierarchically or graphically (e.g., trees, graphs). |
10. Why create Data Structures? | They help organize data efficiently, ensuring optimal performance for operations like searching, inserting, and deleting while facilitating problem-solving through clear relationships among data elements. |
0 Comments