How do I use JavaScript to get the base URL or the URL parameters?
1. Get the Base URL
1. Get the Base URL
The base URL typically consists of the protocol, domain, and optionally the port and path (excluding query parameters and fragments). You can get it using the window.location
object.
// Get the full URL
const fullUrl = window.location.href;
// Get the protocol (e.g., "http:")
const protocol = window.location.protocol;
// Get the host (e.g., "example.com")
const host = window.location.host;
// Get the path (e.g., "/path/to/page")
const pathname = window.location.pathname;
// Combine them to get the base URL
const baseUrl = `${protocol}//${host}${pathname}`;
console.log(baseUrl);
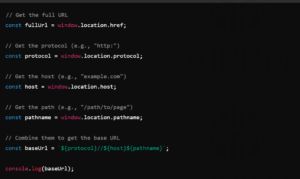
Alternatively, if you only want the base URL without the path:
const baseUrlWithoutPath = `${window.location.protocol}//${window.location.host}`;
console.log(baseUrlWithoutPath);

2. Get URL Parameters
You can get the URL parameters (also called query parameters) using the URLSearchParams
object.
// Create a URLSearchParams object
const params = new URLSearchParams(window.location.search);
// Get a specific parameter by name
const paramValue = params.get('paramName'); // Replace 'paramName' with your parameter key
// Example: If the URL is https://example.com/page?name=John&age=30
console.log(params.get('name')); // Outputs: John
console.log(params.get('age')); // Outputs: 30
// Loop through all parameters
params.forEach((value, key) => {
console.log(`${key}: ${value}`);
});
Example:
If the URL is https://example.com/path/to/page?name=John&age=30
:
- Base URL:
https://example.com/path/to/page
- URL Parameters:
name=John
,age=30