Data structures serve as essential tools for organizing and managing data within a computer’s memory, akin to a toolbelt that keeps various tools in order. To illustrate this concept, consider your own name, which consists of different components: your first name, middle name (if applicable), and last name. Similarly, a bank codeāsuch as an 11-character identifierāencodes specific information where the first four letters denote the bank’s name, the fifth character is typically a zero, and the last six characters identify the particular branch. In this exploration, we will delve into various types of data structures.
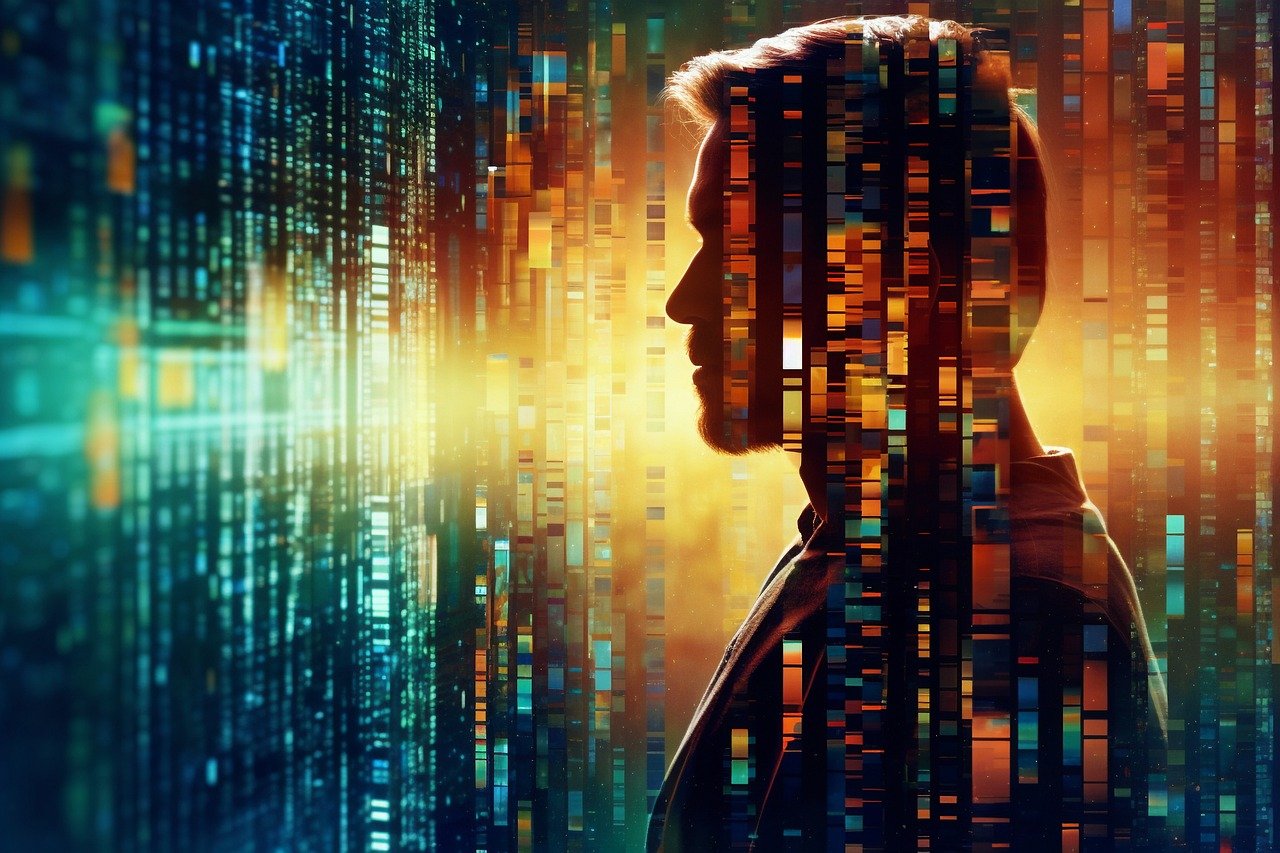
Types of Data Structures
Data structures can be broadly categorized into two main groups:
1. Primitive Data Structures
These structures store data of a single type, including:
- Integers
- Floats
- Characters
- Boolean values (true/false)
2. Non-Primitive Data Structures
These structures can hold multiple types of data and are built from primitive data structures. Examples include:
- Arrays: A collection of elements identified by index.
- Linked Lists: A series of connected nodes where each node contains data and a reference to the next node.
- Trees: Hierarchical structures with nodes connected by edges.
- Graphs: Collections of nodes connected by edges that can represent various relationships.
Arrangement of Data Structures
Data structures are also classified based on their arrangement of data:
1. Linear Data Structures
In these structures, data is arranged sequentially. Examples include:
- Arrays: Fixed-size collections of elements.
- Linked Lists: Dynamic collections where each element points to the next.
Subtypes:
- Static Data Structures: Have a fixed size determined at compile time (e.g., arrays).
- Dynamic Data Structures: Can change size during program execution (e.g., linked lists, stacks).
2. Non-Linear Data Structures
In non-linear structures, data does not follow a sequential order but instead forms a hierarchy or network. Examples include:
- Trees: Comprise nodes with parent-child relationships.
- Graphs: Consist of vertices and edges representing connections between them.
Summary
Understanding these classifications and types of data structures is crucial for efficiently organizing and manipulating data in programming. Each type serves specific purposes and is chosen based on the requirements of the task at hand. By grasping these concepts, one can effectively utilize data structures to enhance algorithm performance and manage large datasets efficiently
Different Types of Data Structures:
1. Arrays
Arrays are fundamental data structures that consist of a collection of elements stored in contiguous memory locations. They can be visualized as a line of boxes, where each box holds a single item. For instance, consider an array that stores the scores of five students:
cint scores[5] = {85, 90, 78, 92, 88};
In this example, scores
refers to the first student’s score (85), scores[1]
to the second student’s score (90), and so on. Accessing elements in an array is very fast because you can directly reference any element using its index. However, arrays have fixed sizes; once created, you cannot easily change their length. If you need to add more elements than the array can hold, you would have to create a new larger array and copy the existing elements over, which can be time-consuming.
2. Linked Lists
Linked lists are linear data structures made up of nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. They can be thought of as a chain of connected blocks. For example:
cstruct Node {
int data;
struct Node* next;
};
struct Node* head = NULL; // Start with an empty list
In this case, each Node
holds an integer and points to the next node. Linked lists allow for easy insertion and deletion of elements since nodes can be added or removed without needing to shift other elements around. However, accessing specific nodes is slower than with arrays because you must traverse the list from the beginning.
3. Stacks
Stacks are dynamic data structures that follow the Last In, First Out (LIFO) principle. You can visualize a stack like a stack of plates: you add new plates on top and remove plates from the top as well. Here’s a simple stack implementation in Python:
pythonstack = []
stack.append('Plate 1') # Add plates
stack.append('Plate 2')
top_plate = stack.pop() # Remove the top plate (Plate 2)
Stacks are useful for scenarios such as backtracking in algorithms or managing function calls in programming.
4. Queues
Queues are linear data structures that operate on the First In, First Out (FIFO) principle. Imagine a line at a grocery store: the first person in line is the first to be served. Hereās how you might implement a queue in Python:
pythonfrom collections import deque
queue = deque()
queue.append('Person A') # Join the queue
queue.append('Person B')
served_person = queue.popleft() # Serve Person A
Queues are ideal for managing tasks that need to be processed in the order they arrive, such as print jobs or customer service requests.
5. Trees
Trees are hierarchical data structures consisting of nodes connected by edges. Each tree has a root node at the top and may have child nodes below it. For example, consider a simple binary tree:
text A
/ \
B C
/ \
D E
In this tree, A
is the root node with two children (B
and C
), and B
has two children (D
and E
). Trees are excellent for representing hierarchical relationships and allow for efficient searching and sorting operations.
6. Heaps
Heaps are specialized tree-based data structures that maintain a specific order based on priority. For instance, in a max-heap, every parent node has a value greater than or equal to its children:
text 10
/ \
9 8
/ \
7 6
Heaps are commonly used in implementing priority queues where tasks are processed based on their priority level.
7. Hash Tables
Hash tables store key-value pairs for fast retrieval based on keys using a hash function. Think of it like an address book where names (keys) point to phone numbers (values). Hereās an example in Python:
pythonphone_book = {}
phone_book['Alice'] = '123-456-7890'
phone_book['Bob'] = '987-654-3210'
alice_number = phone_book['Alice'] # Retrieve Alice's number quickly
Hash tables provide efficient lookups but can face challenges with hash collisions when multiple keys map to the same index.
8. Graphs
Graphs consist of nodes (vertices) connected by edges and can represent various relationships like social networks or city maps. For example:
textA -- B
| \
| C
| /
D
In this undirected graph, nodes represent entities (like people or cities), and edges represent connections between them. Graphs can be directed or undirected, weighted or unweighted, and special algorithms like depth-first search or breadth-first search help traverse them effectively.These data structures serve different purposes and are chosen based on specific requirements such as speed of access, ease of modification, and how data is organized.
Data Structure | Definition | Example | Advantages | Disadvantages |
---|---|---|---|---|
Arrays | A collection of elements stored in contiguous memory locations. | int scores = {85, 90, 78, 92, 88}; | Fast access to elements via index. | Fixed size; difficult to resize. |
Linked Lists | A linear structure made of nodes, each containing data and a reference to the next node. | struct Node { int data; struct Node* next; }; | Easy insertion and deletion of nodes. | Slower access time compared to arrays. |
Stacks | A dynamic structure that follows the Last In, First Out (LIFO) principle. | stack.append('Plate 1'); stack.pop(); | Useful for backtracking and function calls. | Limited access; only the top element can be accessed directly. |
Queues | A linear structure that follows the First In, First Out (FIFO) principle. | queue.append('Person A'); queue.popleft(); | Maintains order of processing tasks. | Limited access; only the front element can be accessed directly. |
Trees | Hierarchical structures with nodes connected by edges; each node may have children. | Binary Tree: A / \ B C | Efficient searching and sorting operations. | Can become unbalanced, affecting performance. |
Heaps | A specialized tree-based structure that maintains a priority order among its elements. | Max-Heap: 10 / \ 9 8 | Efficient for priority queue operations. | More complex to implement than other structures. |
Hash Tables | Stores key-value pairs for fast retrieval based on keys using a hash function. | phone_book['Alice'] = '123-456-7890'; | Fast lookups for large datasets. | Possible hash collisions can slow down performance. |
Graphs | Composed of nodes (vertices) connected by edges; can represent various relationships. | Cities connected by roads: A -- B | Versatile for modeling complex relationships. | Can be complex to traverse and manage. |
This table provides a quick reference to understand each data structure’s characteristics and use cases effectively!